文章目录
- Maven 导入第三方依赖
- JSP中引入
- if条件判断
- for循环输出列表或数组
Maven 导入第三方依赖
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.source>1.7</maven.compiler.source>
<maven.compiler.target>1.7</maven.compiler.target>
<junit.version>4.12</junit.version>
<spring.version>5.2.5.RELEASE</spring.version>
<mybatis.version>3.5.4</mybatis.version>
<mybatis.spring.version>2.0.4</mybatis.spring.version>
<mysql.version>5.1.48</mysql.version>
<commons-dbcp.version>2.7.0</commons-dbcp.version>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>${mybatis.version}</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>${mybatis.spring.version}</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>${mysql.version}</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-dbcp2</artifactId>
<version>${commons-dbcp.version}</version>
</dependency>
<dependency>
<groupId>org.mybatis.generator</groupId>
<artifactId>mybatis-generator-core</artifactId>
<version>1.4.0</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
</dependency>
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
</dependencies>
JSP中引入
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page isELIgnored="false"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<!DOCTYPE html>
<html>
if条件判断
<c:if test="${customer != null}">
<div>
<table>
<tr>
<td class="show_td">用户ID</td>
<td class="show_td">用户名</td>
<td class="show_td">JOBS</td>
<td class="show_td">PHONE</td>
<td class="show_td">Operator</td>
</tr>
<tr>
<td class="show_td">${customer.id}</td>
<td class="show_td">${customer.username}</td>
<td class="show_td">${customer.jobs}</td>
<td class="show_td">${customer.phone}</td>
<td class="show_operator">
<a href="toUpdateCustomer?id=${customer.id}">UPDATE</a>
<a href="">DELETE</a>
</td>
</tr>
</table>
</div>
</c:if>
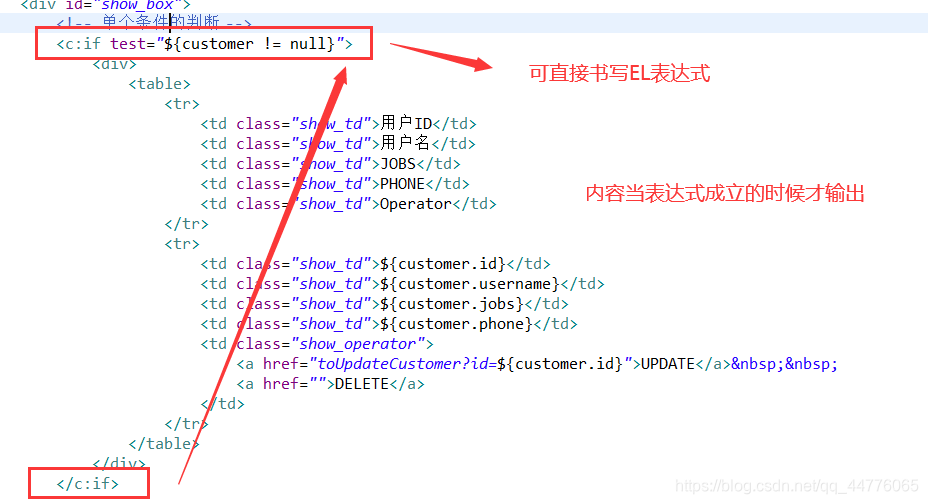
for循环输出列表或数组
<c:if test="${customers != null }">
<div>
<table>
<tr>
<td class="show_td">选择</td>
<td class="show_td">用户ID</td>
<td class="show_td">用户名</td>
<td class="show_td">JOBS</td>
<td class="show_td">PHONE</td>
<td class="show_td">Operator</td>
</tr>
<c:forEach items="${customers}" var="customer">
<tr>
<td class="show_td"><input name="ids" type="checkbox" value="${customer.id}"></td>
<td class="show_td">${customer.id}</td>
<td class="show_td">${customer.username}</td>
<td class="show_td">${customer.jobs}</td>
<td class="show_td">${customer.phone}</td>
<td class="show_operator">
<a href="toUpdateCustomer?id=${customer.id}">UPDATE</a>
<a id="deleteCustomer" href="deleteCustomer?id=${customer.id}" onclick="return confirmDelete()">DELETE</a>
</td>
</tr>
</c:forEach>
<tr>
<td colspan="3" style="text-align: center;"><input type="button" id="deleteButton" value="删除选中项" onclick="deleteCustomers()" ></td>
<td colspan="3" style="text-align: center;"><input type="button" value="修改选中项" onclick="updateCustomers()" ></td>
</tr>
<tr>
<td colspan="2" style="text-align: center;"><a href="pageChange?changePage=-1">上一页</a></td>
<td colspan="2" style="text-align: center;">${sessionScope.currentPage + 1} / ${sessionScope.totalPage}</td>
<td colspan="2" style="text-align: center;"><a href="pageChange?changePage=1">下一页</a></td>
</tr>
</table>
</form>
</div>
</c:if>
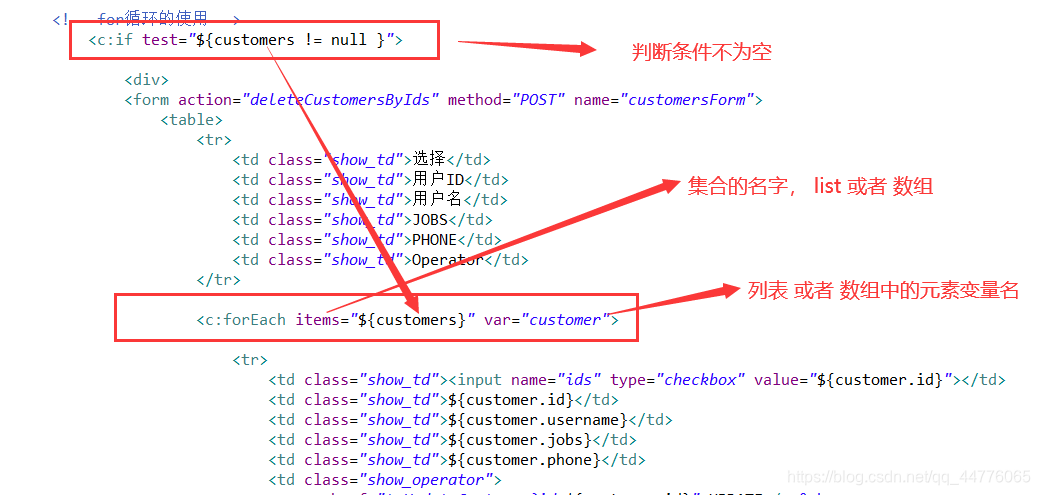